Tips for Optimizing Your Android App’s Performance
- Date July 28, 2023
Performance optimization is essential for ensuring user satisfaction and engagement in the cutthroat app industry of today. The article that follows will discuss a number of suggestions and techniques for improving the functionality and user experience of your Android app.
Efficient Data Handling
Efficiently handling data in your Android app is crucial for optimizing performance and ensuring a smooth user experience. By minimizing network requests, optimizing data caching, using efficient data structures, and implementing lazy loading techniques, you can significantly improve your app’s performance.
A. Minimize network requests:
- Reduce the number of network requests by consolidating data.
- Combine multiple API calls into a single request using batch processing or pagination.
- Implement effective data synchronization strategies to avoid unnecessary data transfers.
B. Optimize data caching:
- Apply caching techniques to data that is often accessed.
- Utilize the caching capabilities provided by libraries like Retrofit or Volley.
- Employ appropriate cache expiration strategies to ensure data freshness.
- Consider using in-memory caching for faster access to frequently used data.
C. Use efficient data structures:
- Choose the right data structure for your specific data handling requirements.
- Use HashMaps or SparseArrays for fast key-value lookups.
- Opt for ArrayLists or LinkedLists based on the usage pattern.
- Consider using efficient data structures like TreeSet or TreeMap for sorted data.
D. Implement lazy loading techniques:
- Load data on-demand rather than preloading everything upfront.
- Implement pagination or infinite scrolling for lists to fetch data in smaller chunks.
- Utilize placeholders or skeleton screens to provide a smooth user experience while data is being loaded.
- Use background threads or asynchronous operations to load data without blocking the UI.
User Interface (UI) Optimization
Optimizing the user interface (UI) of your Android app is essential for delivering a smooth and responsive experience to your users. By focusing on reducing layout hierarchy, optimizing view rendering, implementing view recycling, and leveraging Constraint Layout for complex layouts, you can significantly enhance your app’s UI performance. Let’s explore these tips in detail:
A. Reduce layout hierarchy:
- Simplify your layout structure by minimizing nested views and unnecessary ViewGroup containers.
- Use RelativeLayout or ConstraintLayout to achieve flatter view hierarchies.
- Avoid excessive and nested LinearLayouts, which can lead to performance overhead.
B. Optimize view rendering:
- Use lightweight and optimized UI components whenever possible.
- Replace heavy views with lighter alternatives or custom implementations.
- Precompute and cache complex view calculations to avoid redundant computations during rendering.
- Use the ViewStub class to defer the inflation of complex or heavy views until they are actually needed.
C. Implement view recycling:
- Employ RecyclerView instead of ListView for efficient list or grid-based displays.
- Implement proper ViewHolder pattern to recycle views and minimize the creation of unnecessary view instances.
- Use the DiffUtil class to optimize updates and reduce unnecessary view rebindings.
D. Leverage ConstraintLayout for complex layouts:
- Utilize ConstraintLayout for complex or nested layouts, as it offers superior flexibility and performance.
- Take advantage of constraints and guidelines to optimize view positioning and alignment.
- Use the layout editor tools to create efficient and responsive ConstraintLayouts.
Memory Management
Efficient memory management is crucial for optimizing the performance and stability of your Android app. By avoiding memory leaks, optimizing object allocation, implementing efficient garbage collection, and utilizing tools like the Memory Profiler, you can effectively manage memory usage and enhance your app’s performance. Let’s delve into these memory management tips:
A. Avoid memory leaks:
- Be mindful of long-lived references to objects, such as static variables or holding references in singletons.
- Avoid unnecessary object retention, especially in activities or fragments, by releasing references appropriately.
- Use weak references or weak listeners when necessary to prevent unintentional object retention.
B. Optimize object allocation:
- Minimize object creation within performance-critical sections of your code.
- Reuse objects whenever possible instead of creating new ones repeatedly.
- Consider using object pools or object recycling techniques to minimize the frequency of garbage collection.
C. Implement efficient garbage collection:
- Be cautious with large objects or collections that consume significant memory.
- Avoid unnecessary autoboxing and excessive memory allocation through auto-expanding data structures.
- Use the Java Collection API wisely, considering the memory footprint and performance characteristics of different data structures.
D. Use the Memory Profiler tool:
- Utilize Android Studio’s Memory Profiler to identify memory usage patterns and potential leaks.
- Analyze heap dumps and track memory allocations to pinpoint problematic areas.
- Identify and address memory leaks, excessive object retention, or inefficient memory usage using the profiling data.
Multithreading and Asynchronous Operations
To optimize the performance and responsiveness of your Android app, it is essential to leverage multithreading and asynchronous operations effectively. By employing techniques such as offloading resource-intensive tasks from the main thread, utilizing AsyncTask or ThreadPool Executor for background operations, incorporating parallel processing when suitable, and ensuring proper synchronization of shared resources, you can significantly enhance your app’s efficiency and provide a seamless user experience.
Let’s explore these tips further:
A. Offload heavy tasks from the main thread:
- Perform computationally intensive or time-consuming operations, such as network requests or database queries, in background threads.
- Use AsyncTask, Thread, or Kotlin coroutines to execute tasks off the main UI thread.
- Update the UI with the results of background tasks using appropriate mechanisms like runOnUiThread or LiveData.
B. Utilize AsyncTask or ThreadPoolExecutor for background operations:
- AsyncTask provides a convenient way to perform background operations and update the UI thread.
- ThreadPoolExecutor allows fine-grained control over thread management for parallel processing and efficient resource utilization.
- Consider using Executor frameworks like ExecutorService or Executors.newFixedThreadPool for better thread management.
C. Implement parallel processing where applicable:
- Identify tasks that can be processed in parallel to leverage the capabilities of multicore processors.
- Utilize parallel programming constructs like Java’s Fork/Join framework or Kotlin’s coroutines with multiple dispatchers.
D. Properly synchronize shared resources:
- When multiple threads access and modify shared resources, use synchronization mechanisms like locks, semaphores, or synchronized blocks to prevent data races and maintain data integrity.
- Employ thread-safe data structures or use synchronization primitives like Atomic variables where appropriate.
Battery Life Optimization
Optimizing the battery life of your Android app is crucial for enhancing user satisfaction and ensuring prolonged usage without excessive battery drain. By minimizing background processes, efficiently handling location updates, using alarms and jobs wisely, and optimizing CPU and network usage, you can optimize your app’s energy consumption. Let’s explore these battery life optimization tips:
A. Minimize background processes:
- Limit background activities and services to essential tasks only.
- Avoid continuous polling or frequent background updates unless necessary.
- Use mechanisms like foreground services or JobScheduler for long-running background operations that require user awareness.
B. Efficiently handle location updates:
- Use location updates with the appropriate accuracy level required for your app.
- Adjust the update interval and distance thresholds based on the specific use case to balance accuracy and battery consumption.
- Utilize geofencing or location triggers to optimize location-related tasks by reducing continuous polling.
C. Use alarms and jobs wisely:
- Utilize the AlarmManager or JobScheduler for scheduling tasks at specific times or intervals.
- Use appropriate scheduling strategies to batch similar tasks together and avoid unnecessary wake-ups.
- Consider using Doze mode or App Standby mode for idle or infrequently used features to conserve battery.
D. Optimize CPU and network usage:
- Minimize CPU wake locks to prevent the CPU from staying awake unnecessarily.
- Use efficient algorithms and data structures to reduce CPU usage during processing.
- Optimize network usage by minimizing unnecessary data transfers and utilizing strategies like request batching and compression.
Performance Monitoring and Testing
Monitoring and testing the performance of your Android app is essential for identifying and addressing performance issues proactively. By implementing performance monitoring tools, conducting performance testing, optimizing for various device configurations, and leveraging user feedback, you can continuously improve your app’s performance. Let’s explore these performance monitoring and testing tips:
A. conduct performance testing:
- Perform various types of performance testing, including load testing, stress testing, and endurance testing.
- Simulate realistic user scenarios and usage patterns to evaluate your app’s performance under different conditions.
Utilize tools like Android Profiler, JUnit, or UI Automator for performance testing and profiling.
B. Leverage user feedback:
- Encourage users to provide feedback on performance-related issues.
- Actively monitor user reviews, support tickets, and crash reports for performance-related concerns.
- Use analytics tools to track user engagement and identify potential performance bottlenecks.
Conclusion:
Continuous optimization of your Android app’s performance demands meticulous consideration of multiple factors. A well-optimized app not only brings delight to users but also augments your prospects for triumph in the fiercely competitive app market.
Previous post
The Role of Blockchain in Supply Chain Management: Enhancing Transparency, Traceability, and Efficiency
Next post
Understanding Bias in Artificial Intelligence: Challenges, Impacts, and Mitigation Strategies
You may also like
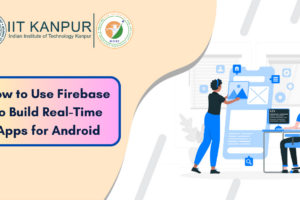
How to Use Firebase to Build Real-Time Apps for Android
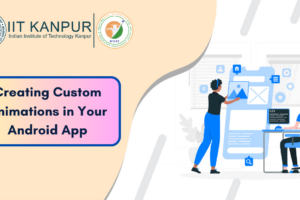
Creating custom animation in your Android app
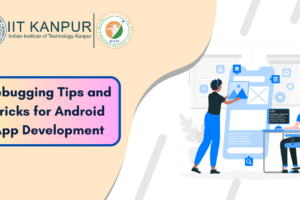