Debugging Tips and Tricks in Android App Development
- Date July 31, 2023
Debugging plays a crucial role in the development of Android applications. It helps developers identify and fix errors, ensuring that the app runs smoothly and delivers an optimal user experience. In this article, we will explore various debugging tips and tricks that can enhance your efficiency and effectiveness as an Android app developer.
Table of Contents
- Introduction to Debugging
- Setting Up the Development Environment
- Logging and Debugging Statements
- Using Breakpoints
- Analyzing Stack Traces
- Debugging with the Android Studio Debugger
- Inspecting Variables and Expressions
- Profiling and Performance Optimization
- Emulator and Device Debugging
- Remote Debugging
- Debugging Network Issues
- Using Third-Party Debugging Tools
- Dealing with Memory Leaks
- Debugging UI and Layout Issues
- Best Practices for Effective Debugging
1. Introduction to Debugging
Debugging is the process of identifying and resolving issues or bugs in software applications. It involves analyzing the code, understanding its execution flow, and identifying the root cause of errors. Effective debugging can significantly reduce development time and ensure the delivery of high-quality apps.
2. Setting Up the Development Environment
Before diving into the debugging process, it’s essential to set up a robust development environment. Make sure you have the latest version of Android Studio installed, along with the necessary SDKs and emulators for testing.
3. Logging and Debugging Statements
One of the simplest yet powerful debugging techniques is logging. By strategically placing log statements in your code, you can track the flow of execution and monitor the values of variables at different stages. Android provides the Log class, which allows you to output logs of different severity levels.
4. Using Breakpoints
Breakpoints are markers in your code that pause the execution at a specific line. They enable you to inspect variables, step through the code, and analyze the program’s state. By setting breakpoints at critical points in your app, you can isolate and fix issues effectively.
5. Analyzing Stack Traces
When an app crashes or encounters an exception, a stack trace is generated. Stack traces provide valuable information about the sequence of method calls leading up to the error. Analyzing stack traces can help you identify the exact location and cause of the exception, making it easier to resolve.
6. Debugging with the Android Studio Debugger
Android Studio provides a powerful debugger that allows you to step through your code, set breakpoints, inspect variables, and analyze the state of your app during runtime. Familiarize yourself with the debugging features offered by Android Studio to leverage its full potential.
7. Inspecting Variables and Expressions
During debugging, it’s essential to examine the values of variables and expressions. Android Studio provides a “Variables” window where you can inspect and modify variable values while the app is paused at a breakpoint. This feature is particularly helpful when trying to understand why a specific value is incorrect or unexpected.
8. Profiling and Performance Optimization
Debugging is not only about fixing bugs but also about optimizing your app’s performance. Use the profiling tools in Android Studio to analyze CPU usage, memory allocations, and network activity. By identifying performance bottlenecks, you can optimize your code and enhance the overall user experience.
9. Emulator and Device Debugging
Android Studio allows you to debug your app on both emulators and physical devices. While emulators provide a convenient testing environment, debugging on real devices can help uncover device-specific issues. Test your app on a variety of devices to ensure compatibility and address any device-specific bugs.
10. Remote Debugging
Remote debugging enables you to debug an app running on a remote device or emulator. It’s particularly useful when testing on devices with limited physical access or debugging apps deployed on user devices. Android Studio supports remote debugging via Wi-Fi or ADB over network connections.
11. Debugging Network Issues
Mobile apps often rely on network requests to fetch data from servers. Debugging network issues involves analyzing API requests, responses, and network traffic. Android Studio’s network profiler can help you track network activity, identify slow requests, and troubleshoot connectivity problems.
12. Using Third-Party Debugging Tools
In addition to the built-in debugging features of Android Studio, there are several third-party tools available that can enhance your debugging workflow. These tools offer advanced debugging capabilities, such as memory leak detection, method profiling, and UI inspection.
13. Dealing with Memory Leaks
Memory leaks can lead to performance degradation and app crashes. Android Studio provides tools like the Memory Profiler to detect and analyze memory leaks in your app. By identifying objects that are not properly released, you can free up memory resources and improve overall app stability.
14. Debugging UI and Layout Issues
UI and layout issues can cause visual glitches and misalignments in your app. Android Studio’s Layout Inspector allows you to inspect the hierarchy and properties of UI components during runtime. This tool helps identify layout issues and facilitates UI debugging for a polished user interface.
15. Best Practices for Effective Debugging
To make the most of your debugging efforts, consider the following best practices:
- Keep your code modular and well-organized.
- Write meaningful comments and documentation.
- Test your app regularly during development.
- Use version control to track changes and revert if necessary.
- Collaborate with other developers and seek assistance when needed.
- Stay updated with the latest Android development tools and techniques.
Conclusion
Debugging is an integral part of the Android app development process. By following the tips and tricks outlined in this article, you can streamline your debugging workflow and ensure the delivery of high-quality apps. Remember to leverage the debugging features provided by Android Studio and explore third-party tools for enhanced capabilities. With effective debugging, you can create robust and user-friendly Android applications.
FAQs
- What is debugging in Android app development? Debugging in Android app development refers to the process of identifying and fixing errors or bugs in software applications. It involves analyzing the code, understanding its execution flow, and resolving issues to ensure smooth app performance.
- What are some common debugging techniques for Android app development? Common debugging techniques for Android app development include logging and debugging statements, breakpoints, stack trace analysis, using the Android Studio debugger, inspecting variables and expressions, and profiling for performance optimization.
- How can I debug network issues in my Android app? To debug network issues in your Android app, you can use Android Studio’s network profiler, which helps track network activity, analyze API requests and responses, identify slow requests, and troubleshoot connectivity problems.
- Are there any third-party debugging tools available for Android app development? Yes, there are several third-party debugging tools available for Android app development. These tools provide advanced debugging capabilities such as memory leak detection, method profiling, UI inspection, and more.
- What are some best practices for effective debugging? Some best practices for effective debugging include writing modular and well-organized code, regularly testing the app during development, using version control, collaborating with other developers, and staying updated with the latest tools and techniques in Android development.
Previous post
The Regulatory Landscape of Cryptocurrency: Balancing Innovation and Security
You may also like
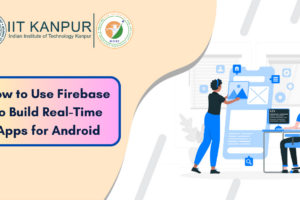
How to Use Firebase to Build Real-Time Apps for Android
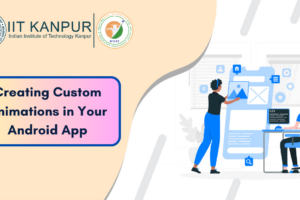